Roll ads are displayed as short videos or images, before, during, or after the video content is played.
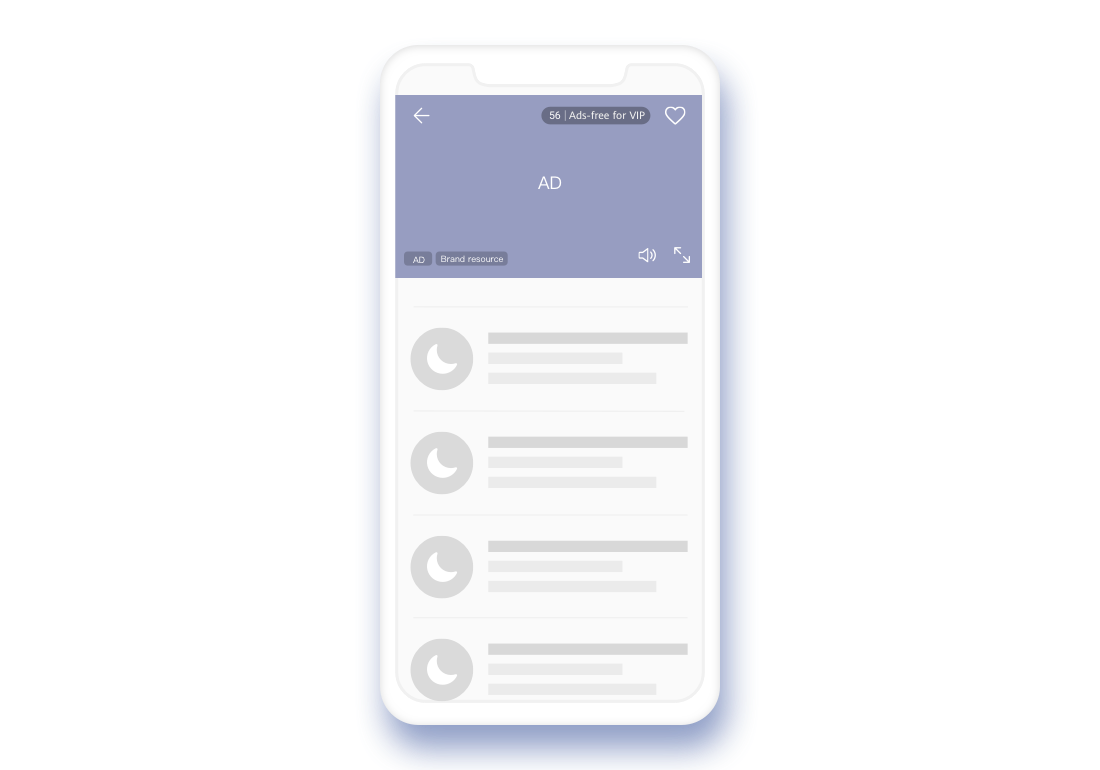
Adding Roll Ads
- Build an InstreamAdLoader object.
The
InstreamAdLoader class provides the
InstreamAdLoader.Builder class for setting the ad unit ID, customizing attributes, and building an
InstreamAdLoader object. The sample code is as follows:
// "testy3cglm3pj0" is the ID of a dedicated test ad unit. Before releasing your app, replace the test ad unit ID with the formal one.
InstreamAdLoader.Builder builder = new InstreamAdLoader.Builder(context, "testy3cglm3pj0");
// Set the maximum total duration of roll ads.
InstreamAdLoader adLoader = builder.setTotalDuration(totalDuration)
// Set the maximum number of roll ads that can be loaded.
.setMaxCount(maxCount)
.setInstreamAdLoadListener(new InstreamAdLoadListener() {
@Override
public void onAdLoaded(List<InstreamAd> ads) {
// Called when an ad is loaded successfully.
...
}
@Override
public void onAdFailed(int errorCode) {
// Called when an ad fails to be loaded.
...
}
}).build();
// "testy3cglm3pj0" is the ID of a dedicated test ad unit. Before releasing your app, replace the test ad unit ID with the formal one.
val builder = InstreamAdLoader.Builder (context, "testy3cglm3pj0")
// Set the maximum total duration of roll ads.
adLoader = builder.setTotalDuration(totalDuration)
// Set the maximum number of roll ads that can be loaded.
.setMaxCount(maxCount)
.setInstreamAdLoadListener(object : InstreamAdLoadListener {
override fun onAdLoaded(ads: List<InstreamAd>) {
// Called when an ad is loaded successfully.
...
}
override fun onAdFailed(i: Int) {
// Called when an ad fails to be loaded.
...
}
}).build()
说明
- You need to set the maximum total duration and maximum number of roll ads based on the length of a video. Petal Ads will return roll ads according to your settings.
For example, if the maximum total duration is 60 seconds and the maximum number of roll ads is 8, at most four 15-second roll ads or two 30-second roll ads will be returned. If the maximum total duration is 120 seconds and the maximum number of roll ads is 4, no more roll ads will be returned after whichever is reached.
- For details, please refer to Android Result Codes.
- Load an ad.
InstreamAdLoader provides the loadAd() method for ad loading.
AdParam is the only parameter. The sample code is as follows:
AdParam.Builder builder = new AdParam.Builder();
// (Optional) Set the parameters for a real-time bidding ad unit.
BiddingParam biddingParam = new BiddingParam();
String slotId = "testy3cglm3pj0";
builder.addBiddingParamMap(slotId, biddingParam);
builder.setTMax(500);
builder.setCur("Currency code list");
adLoader.loadAd(new AdParam.Builder().build());
adLoader!!.loadAd(AdParam.Builder().build())
After loadAd() is called, the listener receives a callback on success or failure.
说明
Before reusing InstreamAdLoader to load ads, ensure that the previous request is complete.
- Display roll ads.
After roll ads are loaded, you need to display them in the listener callback method. The "Why this ad" icon (i) or "Dislike this ad" icon (x), and the ad flag must be displayed in the corner of each ad view.
The procedure for displaying a roll ad is as follows:
- Define the roll ad layout.
Customize a layout to display the ad assets in InstreamAd.
<RelativeLayout
android:id="@+id/instream_ad_container"
android:layout_width="match_parent"
android:layout_height="200dp"
android:visibility="gone">
<!-- Roll ad view. -->
<com.huawei.hms.ads.instreamad.InstreamView
android:id="@+id/instream_view"
android:layout_width="match_parent"
android:layout_height="match_parent" />
<!-- Skip button view. -->
<TextView
android:id="@+id/instream_skip"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
...
/>
<!-- Countdown view. -->
<TextView
android:id="@+id/instream_count_down"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
...
/>
<!-- Ad flag view. -->
<TextView
android:id="@+id/instream_ad_flag"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
...
/>
<!-- Why this ad view. -->
<ImageView
android:id="@+id/instream_why_this_ad"
android:layout_width="14dp"
android:layout_height="14dp"
...
/>
<!-- Click a button. -->
<TextView
android:id="@+id/instream_call_to_action"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
...
/>
</RelativeLayout>
说明
You need to design the roll ad layout based on your video playback layout, such as the width and height of the roll ad layout and the position of each view control.
- Register ads with the ad view.
After the
InstreamAd object list is obtained, register ads with the ad view. The sample code is as follows:
instreamView.setInstreamAds(ads);
instreamView!!.setInstreamAds(ads)
- Listen for ad status.
InstreamMediaStateListener,
InstreamMediaChangeListener, and
MediaMuteListener are provided to listen for roll ad status. The sample code is as follows:
instreamView.setInstreamMediaChangeListener(new InstreamMediaChangeListener(){
@Override
public void onSegmentMediaChange(InstreamAd ad) {
// Switch from one roll ad to another.
...
}
});
instreamView.setInstreamMediaStateListener(new InstreamMediaStateListener() {
@Override
public void onMediaProgress(int percent, int playTime) {
// Playback process.
...
}
@Override
public void onMediaStart(int playTime) {
// Start playback.
...
}
@Override
public void onMediaPause(int playTime) {
// Pause playback.
...
}
@Override
public void onMediaStop(int playTime) {
// Stop playback.
...
}
@Override
public void onMediaCompletion(int playTime) {
// Playback is complete.
...
}
@Override
public void onMediaError(int playTime, int errorCode, int extra) {
// Playback error.
...
}
});
instreamView.setMediaMuteListener(new MediaMuteListener() {
@Override
public void onMute() {
// Mute a roll ad.
...
}
@Override
public void onUnmute() {
// Unmute a roll ad.
...
}
});
instreamView!!.setInstreamMediaChangeListener(InstreamMediaChangeListener {
// Switch from one roll ad to another.
...
})
instreamView!!.setInstreamMediaStateListener(object : InstreamMediaStateListener {
override fun onMediaProgress(i: Int, i1: Int) {
// Playback process.
...
}
override fun onMediaStart(i: Int) {
// Start playback.
...
}
override fun onMediaPause(i: Int) {
// Pause playback.
...
}
override fun onMediaStop(i: Int) {
// Stop playback.
...
}
override fun onMediaCompletion(i: Int) {
// Playback is complete.
...
}
override fun onMediaError(i: Int, i1: Int, i2: Int) {
// Playback error.
...
}
})
instreamView!!.setMediaMuteListener(object : MediaMuteListener {
override fun onMute() {
// Mute a roll ad.
...
}
override fun onUnmute() {
// Unmute a roll ad.
...
}
})
- Destroy an ad.
When the display of a roll ad ends, you should call the
destroy() method to destroy the roll ad view. The sample code is as follows:
Obtaining the Parameters of a Real-Time Bidding Ad Unit
注意
The API for obtaining the parameters of a real-time bidding ad unit needs to be called after the ad is loaded. Since the BiddingInfo object might be null, you need to check for null first before proceeding with any bidding-related operations.
Parameter object BiddingInfo
Code for obtaining the object:
// "testy3cglm3pj0" is the ID of a dedicated test ad unit. Before releasing your app, replace the test ad unit ID with the formal one.
InstreamAdLoader.Builder builder = new InstreamAdLoader.Builder(context, "testy3cglm3pj0");
InstreamAdLoader adLoader = builder.
.setInstreamAdLoadListener (new InstreamAdLoadListener() {
@Override
public void onAdLoaded(List<InstreamAd> ads) {
// Called when an ad is loaded successfully.
for (InstreamAd ad : ads) {
BiddingInfo biddingInfo = ad.getBiddingInfo();
if ( biddingInfo != null ) {
// Handle bidding-related operations.
}
}
...
}
}).build();
Displaying Advertiser Information
When a roll ad delivers advertiser information, the advertiser information icon needs to be rendered. When a user clicks the icon, an API of the SDK will be called to display the advertiser information dialog box.
Sample code:
// advertiserIcon is an advertiser information icon rendered by yourself.
...
advertiserIcon.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if (instreamAd.hasAdvertiserInfo()) {
instreamView.showAdvertiserInfoDialog(advertiserIcon, true);
}
}
});
...
// When a roll ad is switched, determine whether to deliver the advertiser information. If not, hide the rendered advertiser information dialog box.
instreamView.setInstreamMediaChangeListener(new InstreamMediaChangeListener() {
@Override
public void onSegmentMediaChange(InstreamAd ad) {
Log.i(TAG, "onSegmentMediaChange");
if (null != ad && !ad.hasAdvertiserInfo()) {
advertiserIcon.setVisibility(View.GONE); // Hide the advertiser information icon when no advertiser information is delivered.
}
}
});
// advertiserIcon is an advertiser information icon rendered by yourself.
...
advertiserIcon.setOnClickListener(View.OnClickListener {
if (instreamAd.hasAdvertiserInfo()) {
instreamView.showAdvertiserInfoDialog(advertiserIcon, true)
}
})
instreamView!!.setInstreamMediaChangeListener { ad ->
Log.i(FragmentActivity.TAG, "onSegmentMediaChange")
if (null != ad && !ad.hasAdvertiserInfo()) {
advertiserIcon.setVisibility(View.GONE) // Hide the advertiser information icon when no advertiser information is delivered.
}
}
Testing Roll Ads
When testing roll ads, use the dedicated test ad unit ID to load test ads. This avoids invalid ad clicks during the test.
The test ad unit ID is used only for function commissioning. Before releasing your app, access the official website of Petal Ads Publisher Service and click Start now. Sign in to Petal Publisher Center, apply for a formal ad unit ID, and replace the test ad unit ID with the formal one. For details, please refer to Adding/Enabling an Ad Unit.
The following table lists the dedicated ad unit ID for roll ad testing.
Ad Format | Ad Type | Dimensions in px (W x H) | Test Ad Unit ID |
Roll | Image or video | 640 x 360 | testy3cglm3pj0 |
After you download the sample code for roll ads and execute the code, the following screen will be displayed.
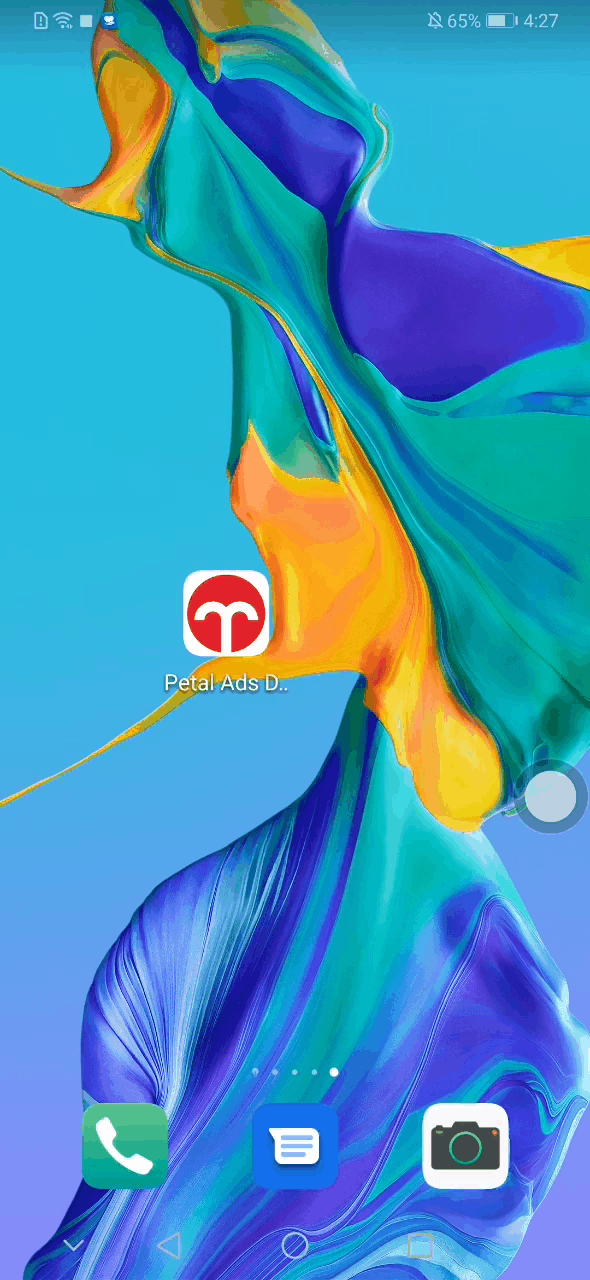
You can also learn how to integrate roll ads based on the corresponding codelab.