Mobile Phone Themes
GUI
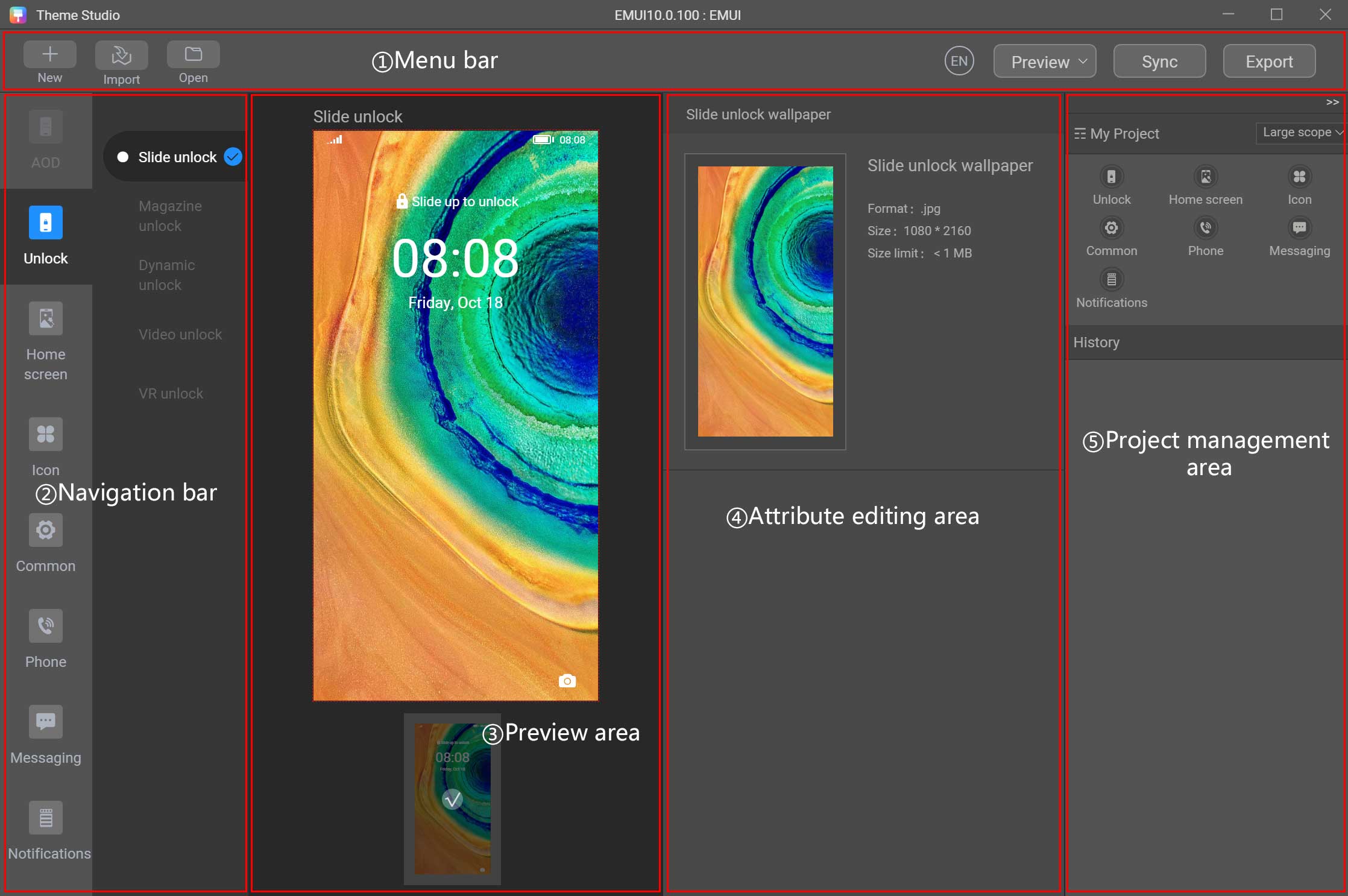
1. Menu bar
2. Navigation bar
3. Preview area
4. Attribute editing area
5. Project management area
Creating a Theme
- Click New Project, enter a project name, select a project type, and click Confirm.
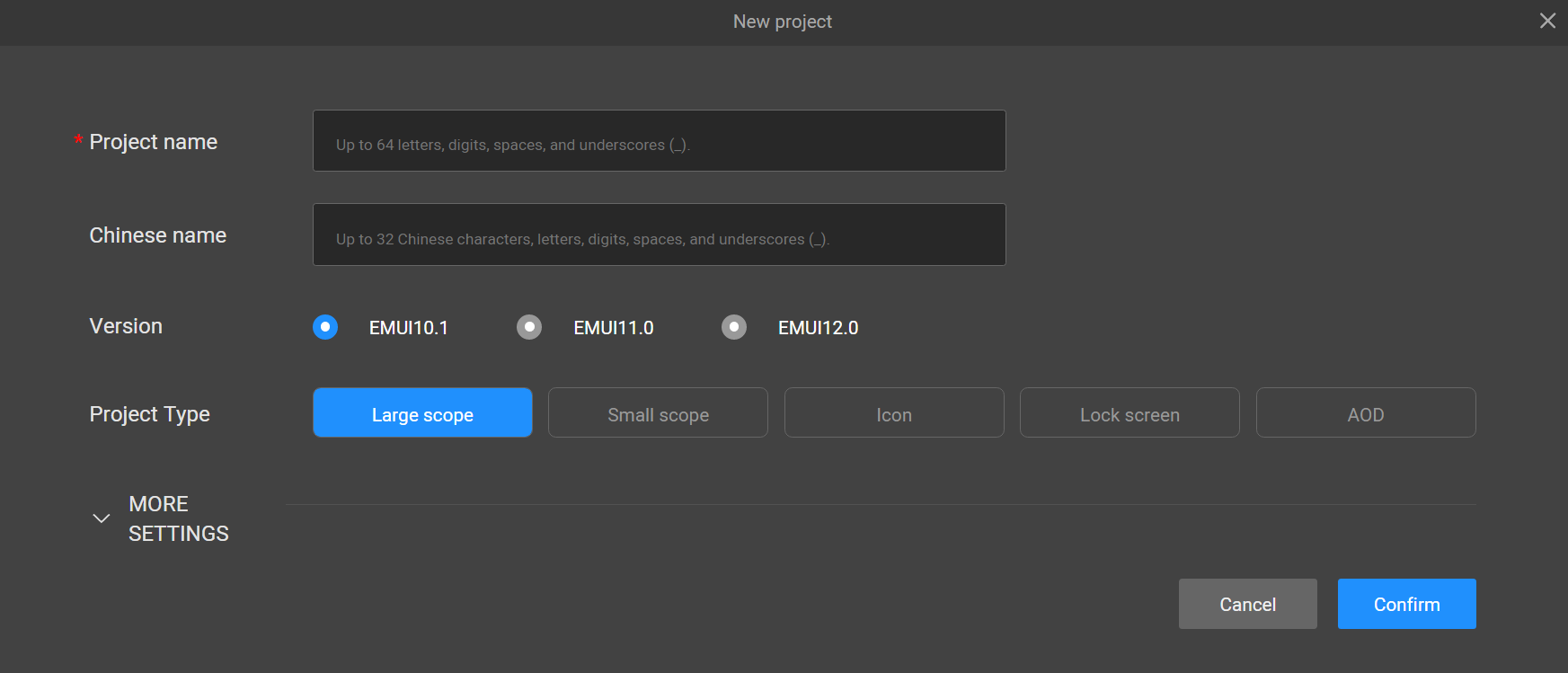
- According to the selected theme type, click any function icon on the navigation bar and configure related options in the editing area. When completing the configuration, you can view the theme effects in the preview area.
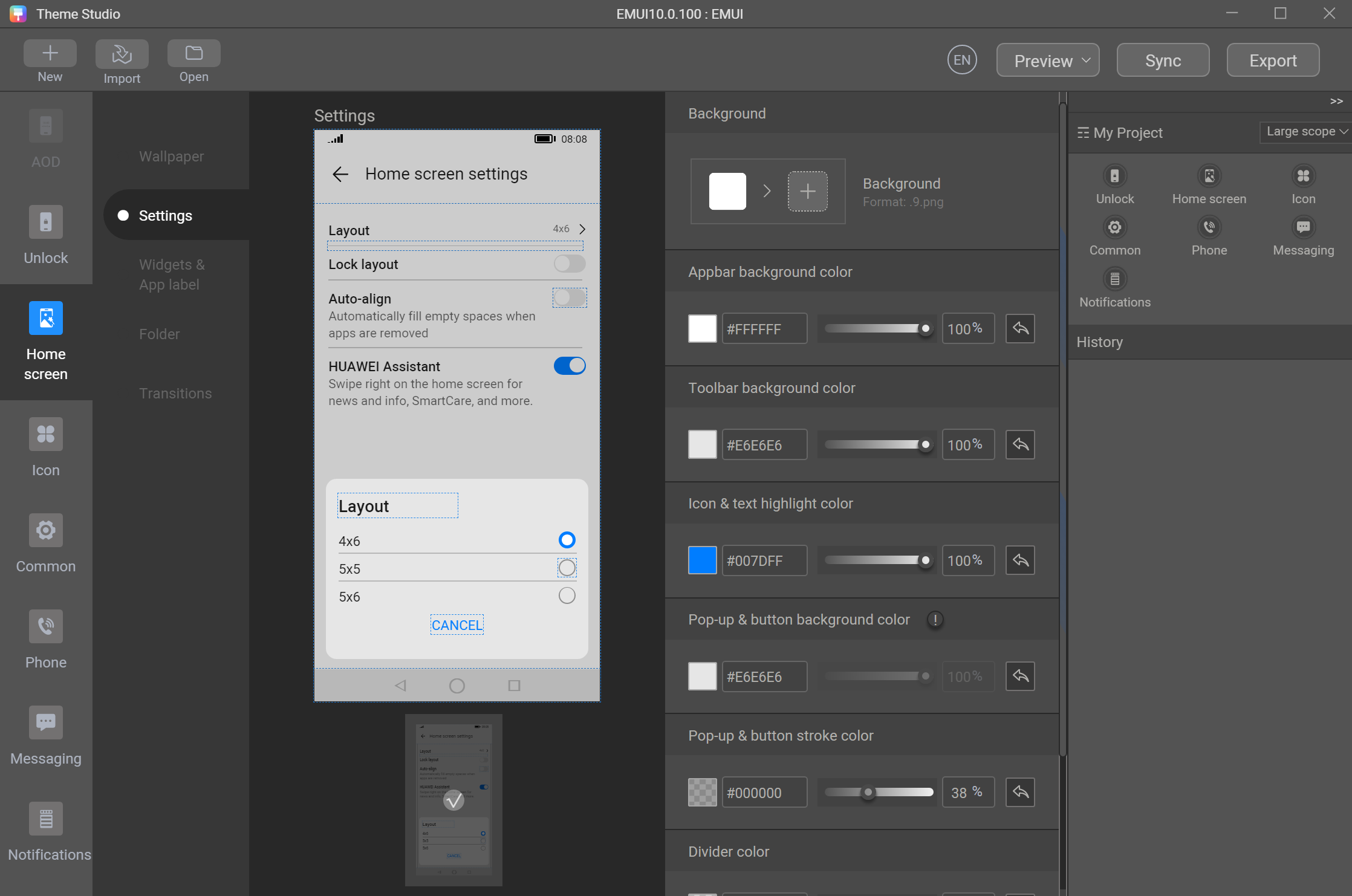
Synchronizing the Theme
After finishing all modules of the theme, connect your Huawei phone to the local PC and enable USB debugging on the phone. Click Sync on the menu bar to synchronize the edited theme to the phone. After the synchronization is successful, you will be able to select the theme in the Themes app.
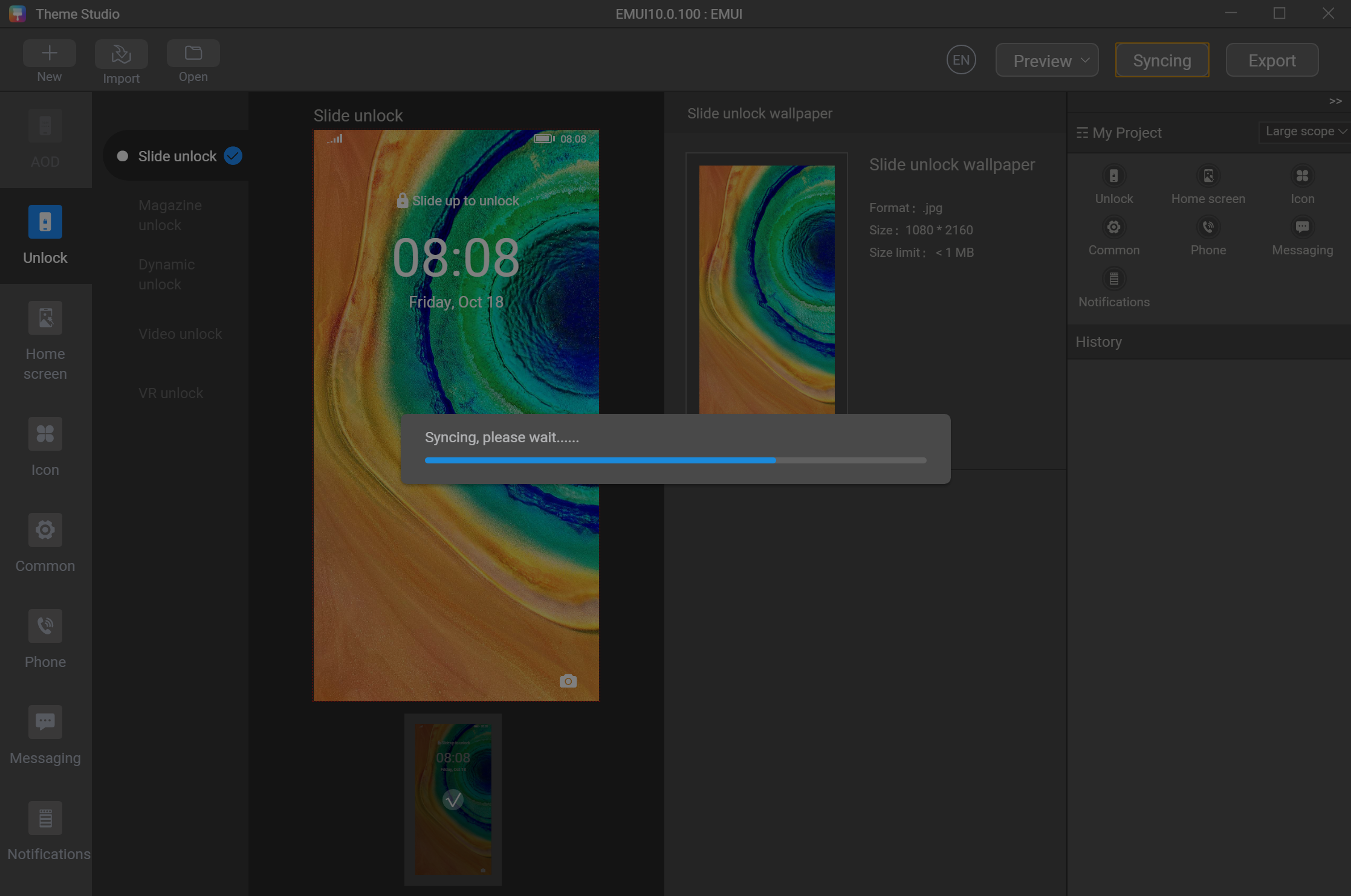
Exporting a Theme
After checking the theme, click Export on the menu bar to export the theme.
- Check or set the preview image.
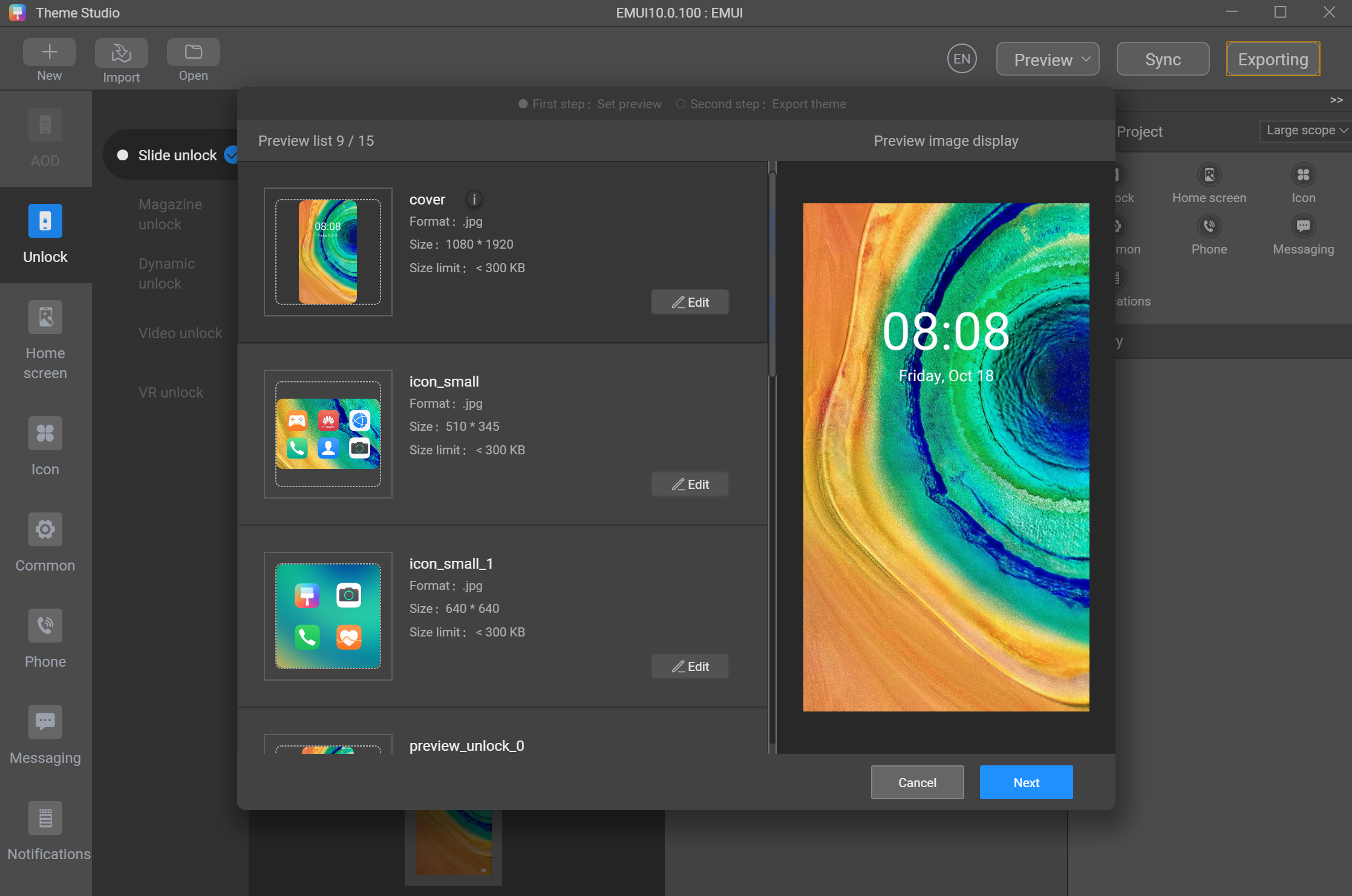
- Enter details about the theme, confirm the information, and click Export.
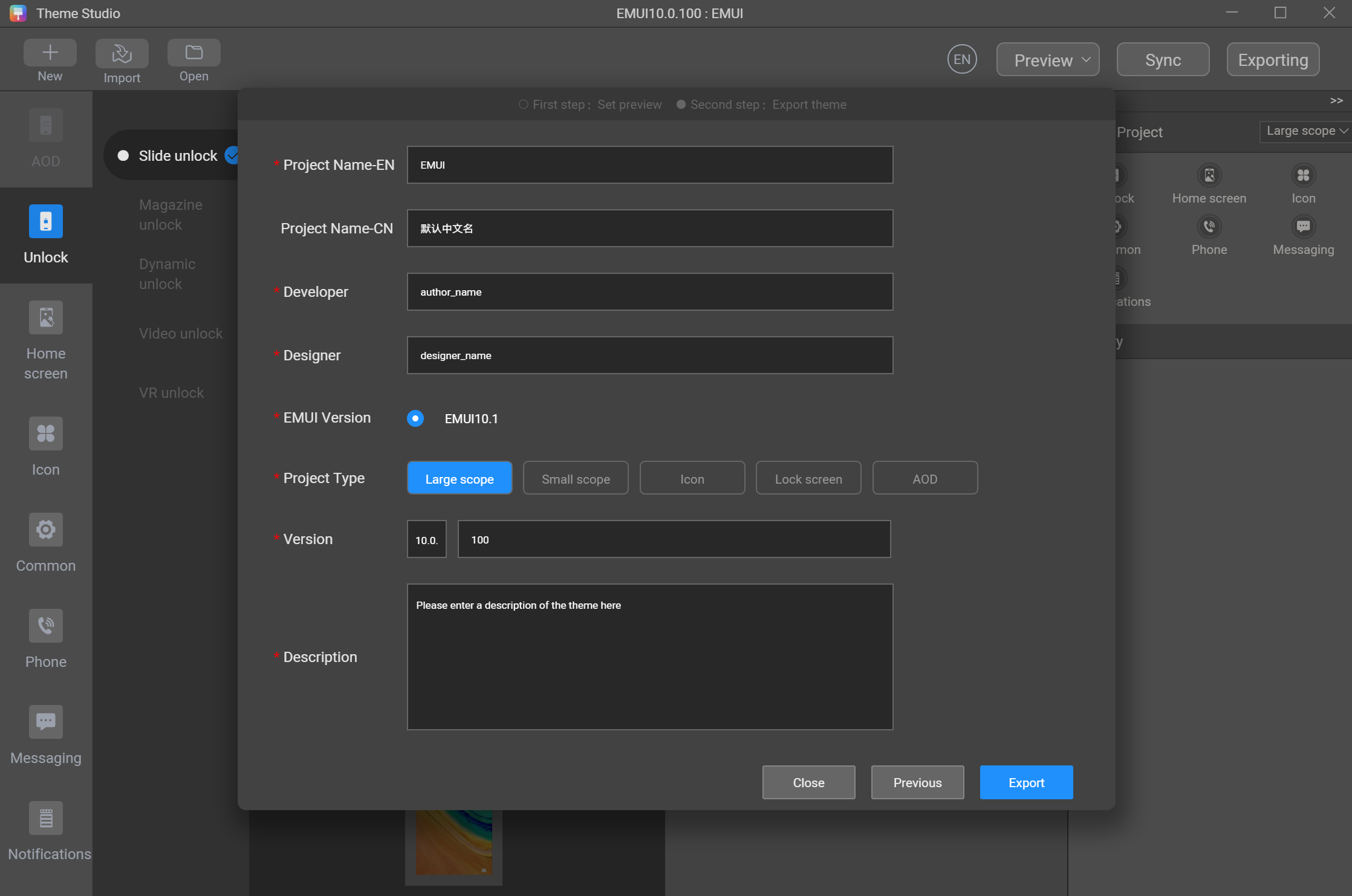
Importing a Theme
Click Import, select the .hwt theme file, and import it.
- For a new project
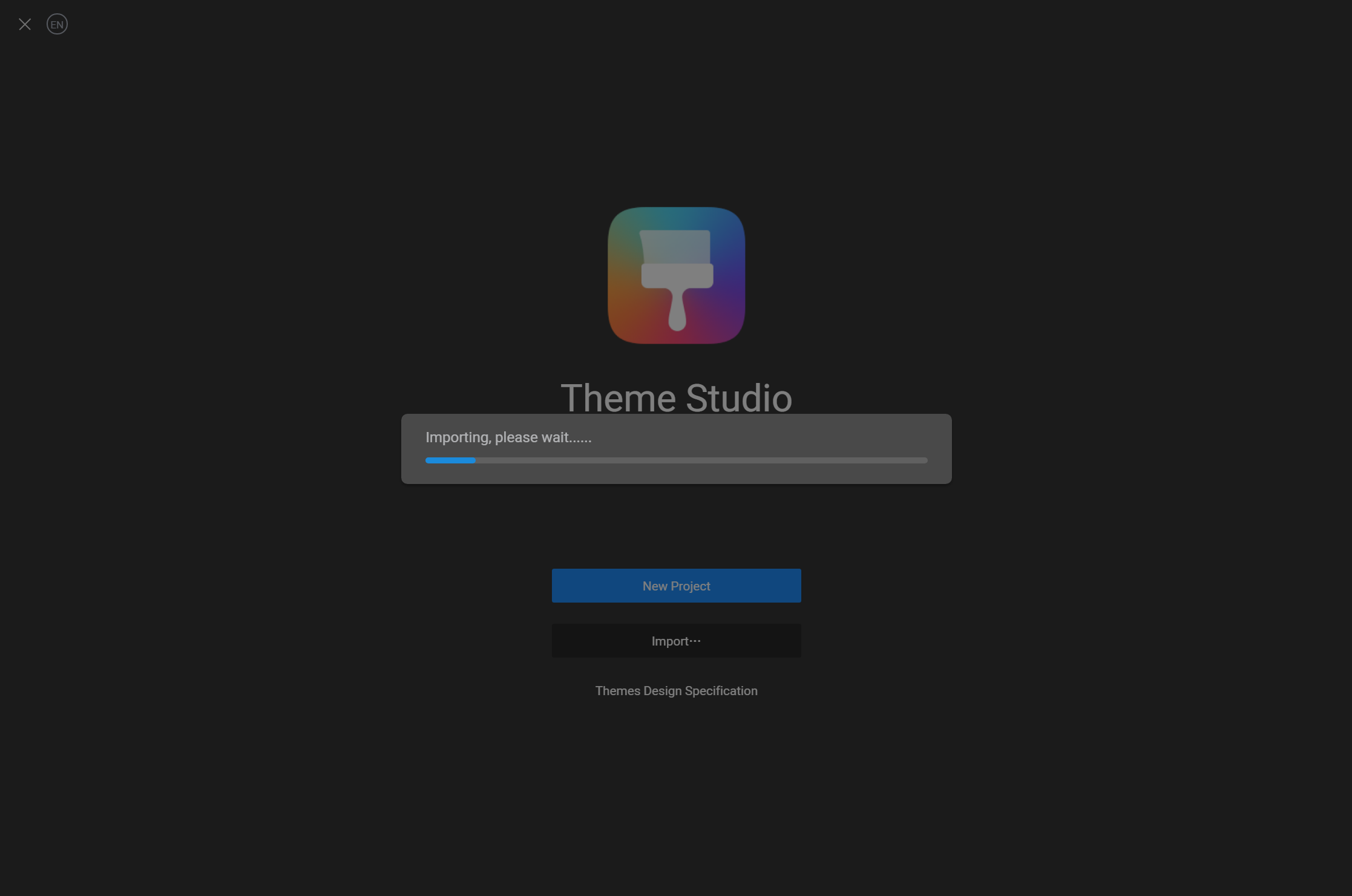
- For an earlier project
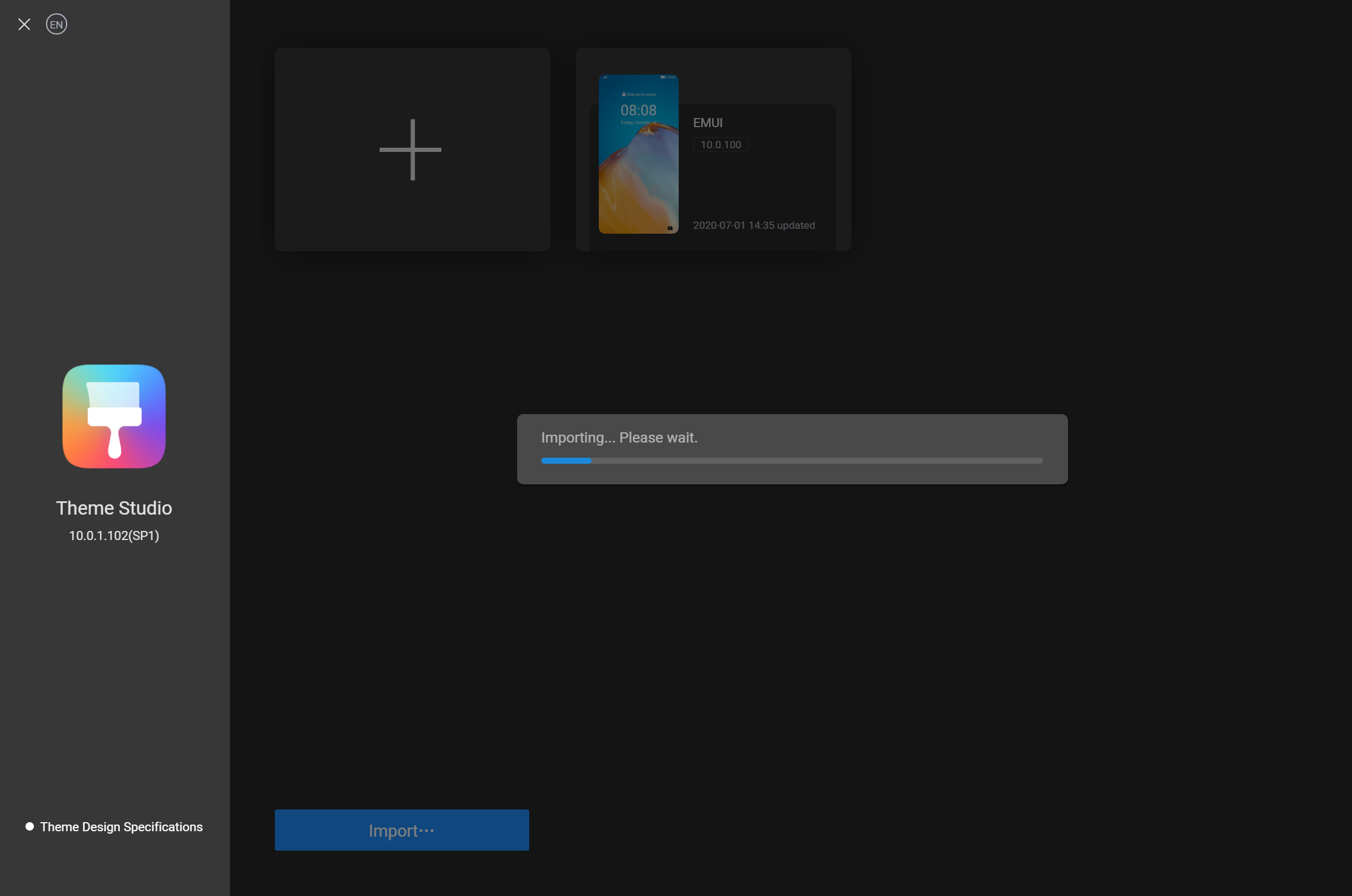
Watch Face Themes
GUI
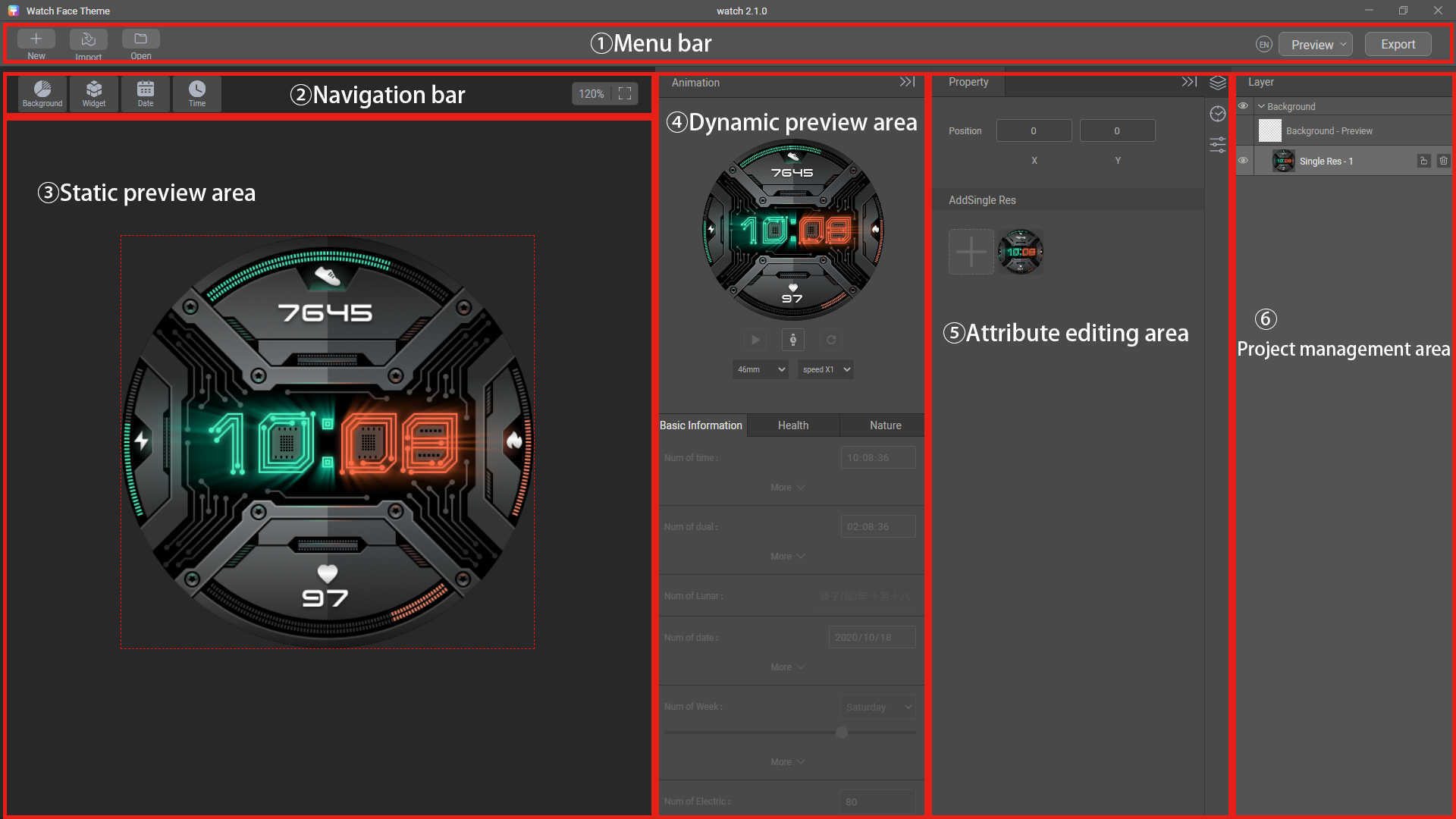
1. Menu bar
2. Navigation bar
3. Static preview area
4. Dynamic preview area
5. Attribute editing area
6. Project management area
Creating a Theme
- Click New Project, enter a watch face name, select a resolution, and click Confirm to create a project.
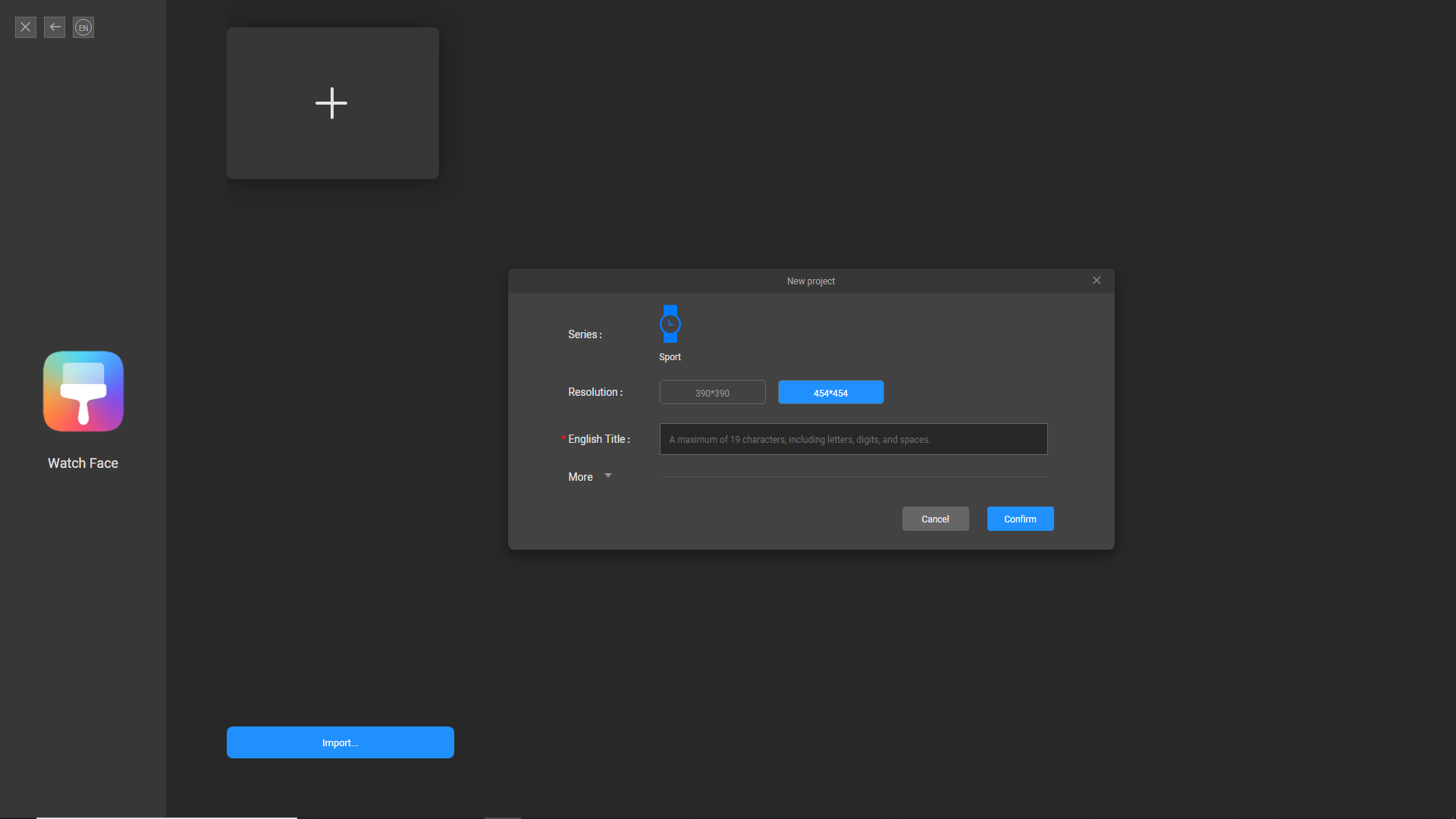
- According to the selected resolution, click any function icon on the navigation bar and configure related options in the editing area. When completing the configuration, you can view the watch face effects in the dynamic preview area.
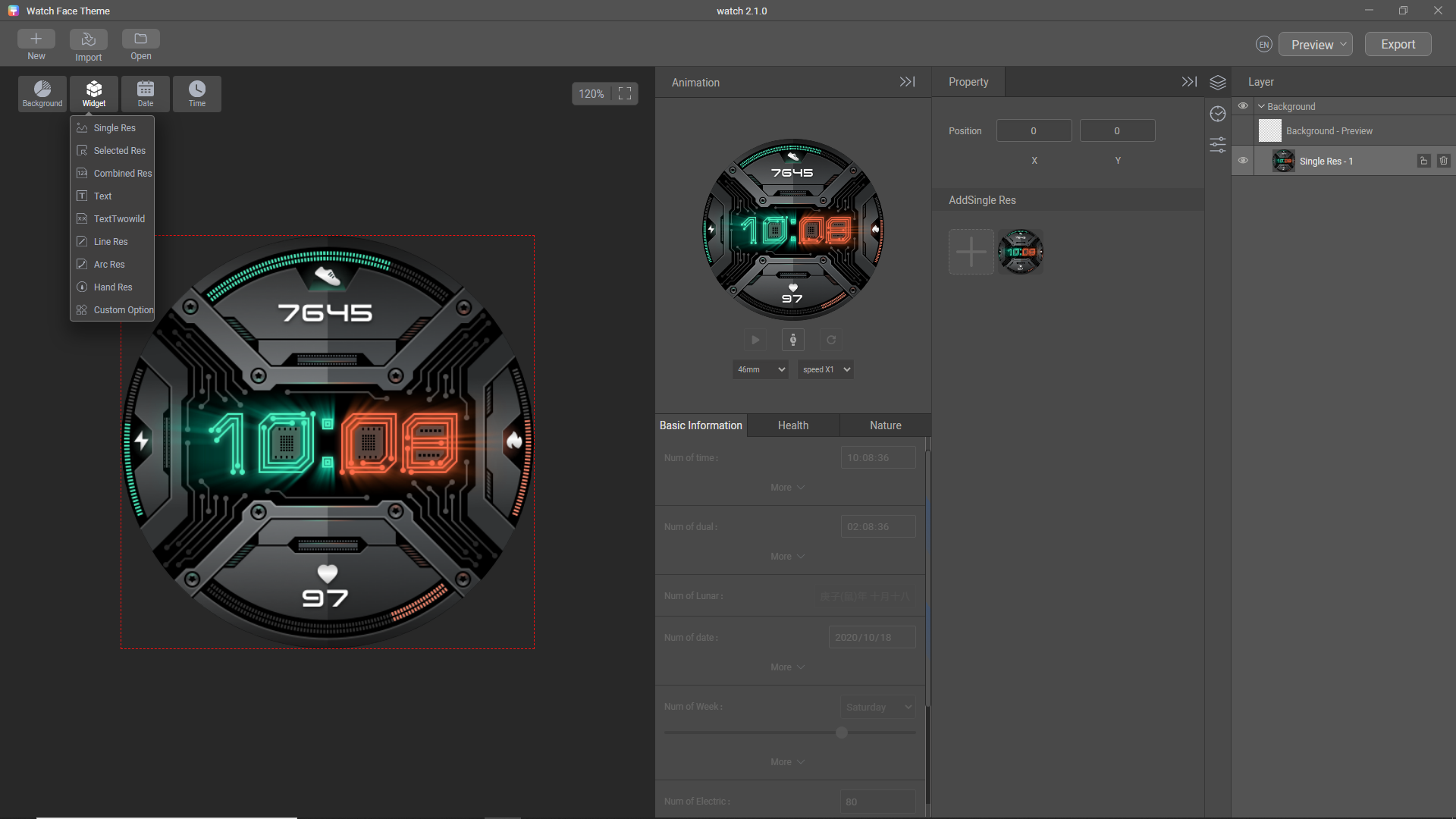
Exporting a Theme
- After checking the watch face, click Export on the menu bar to export the watch face.
- Check or set the preview image.
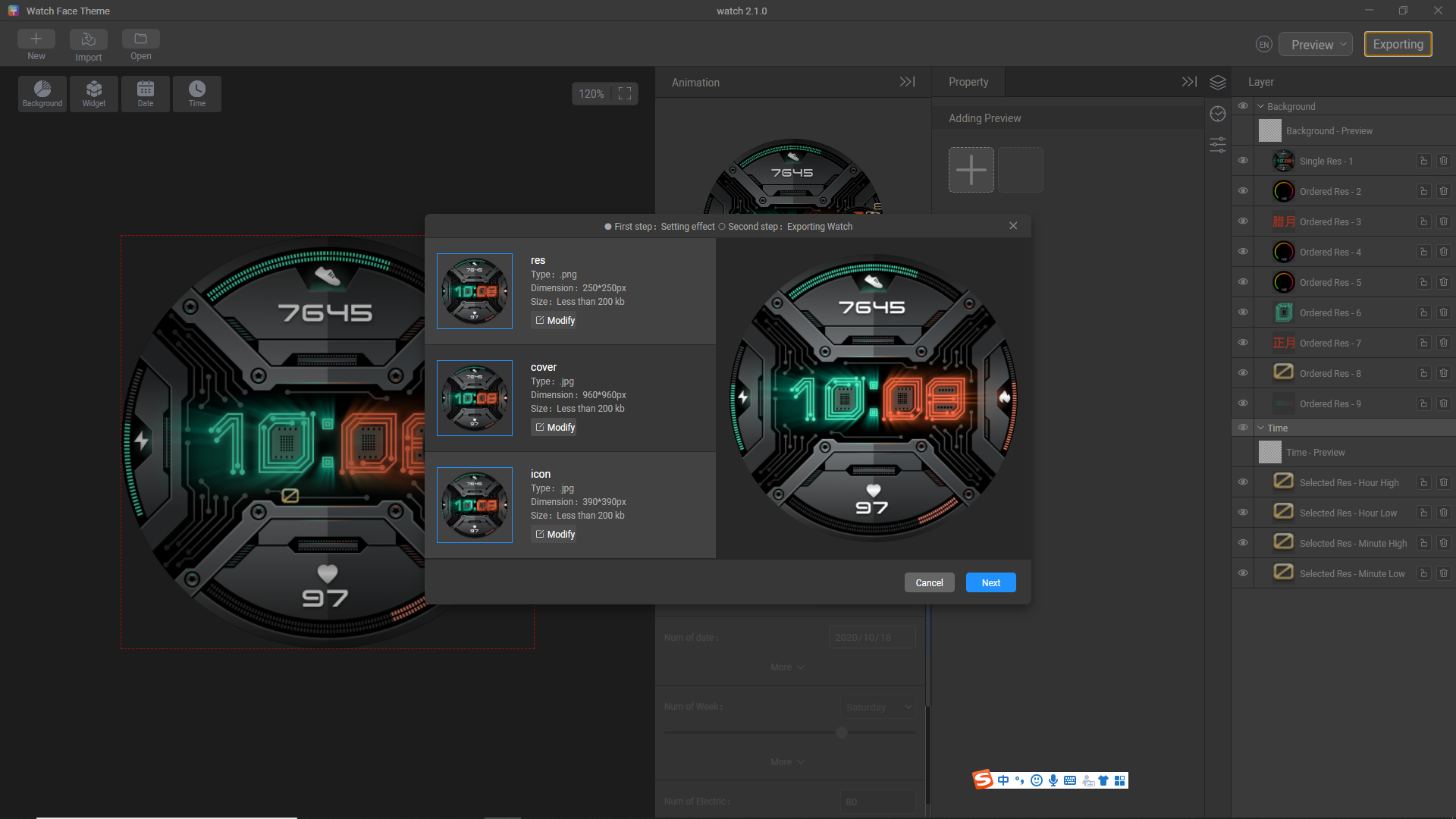
- Configure watch face information, and click Export.
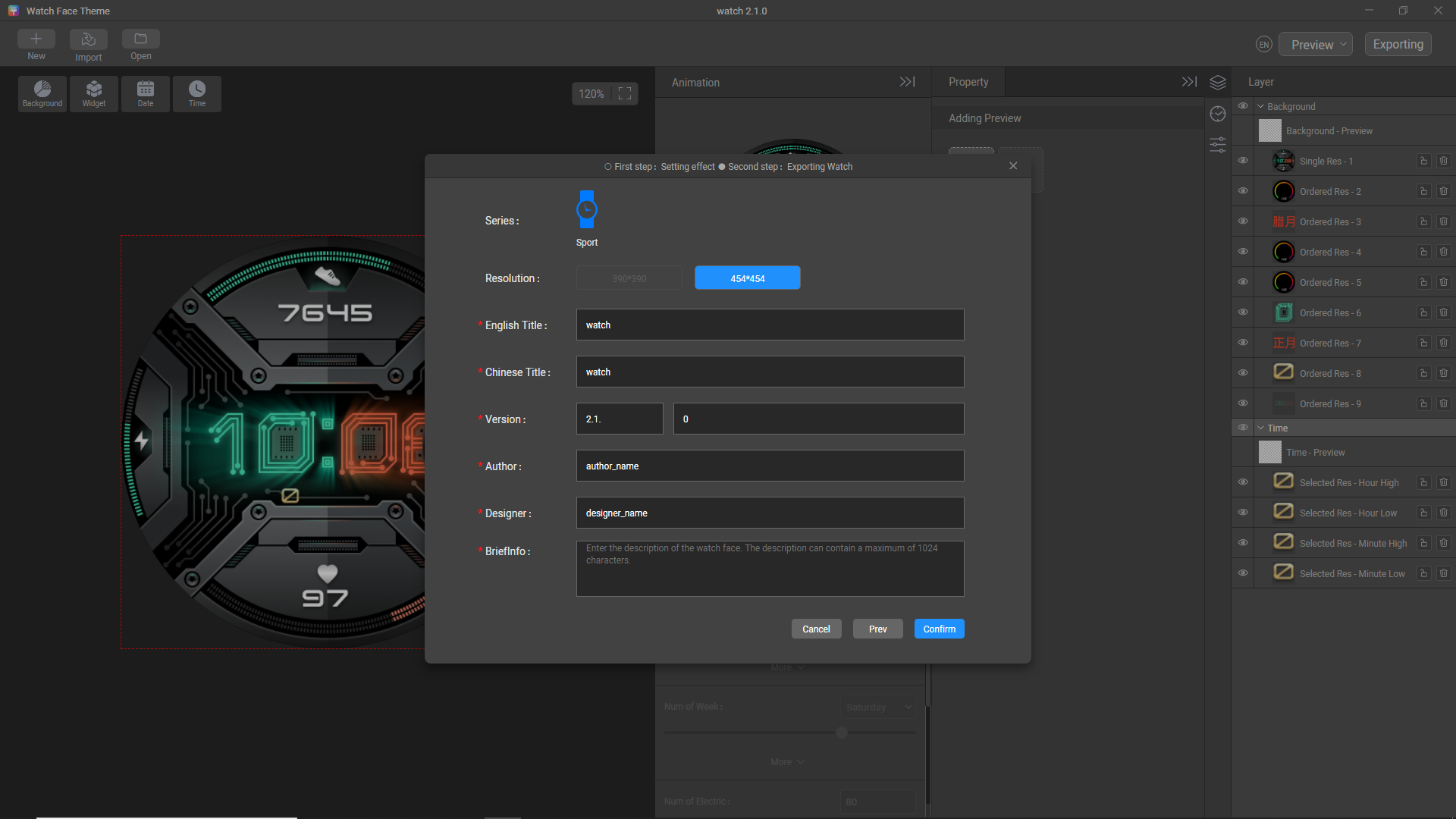
Importing a Theme
Click Import, select the .hwt watch face file (the file name extension of the exported .zip file is changed to .hwt), and import the watch face.
- For a new project
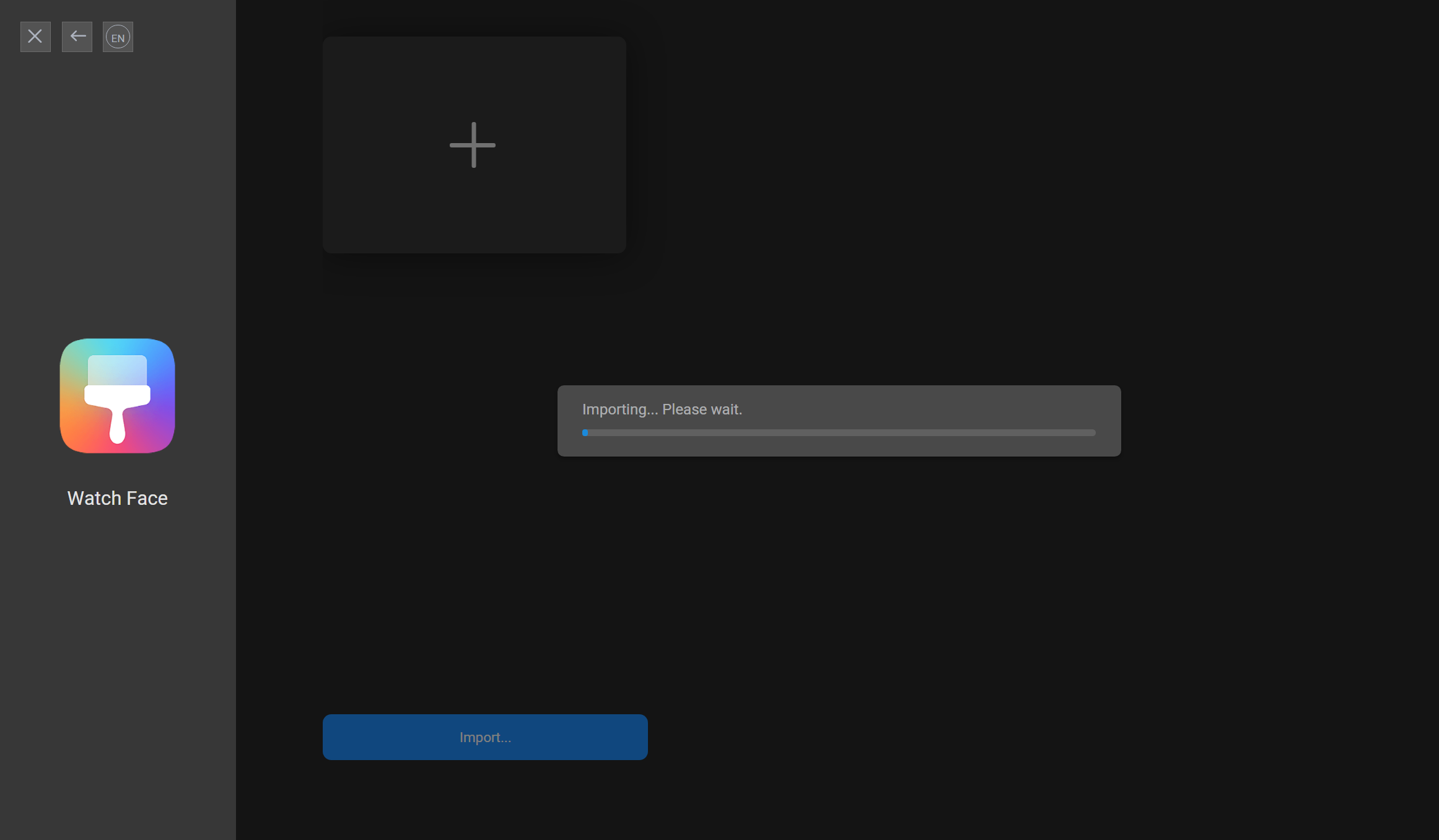
- For an earlier project
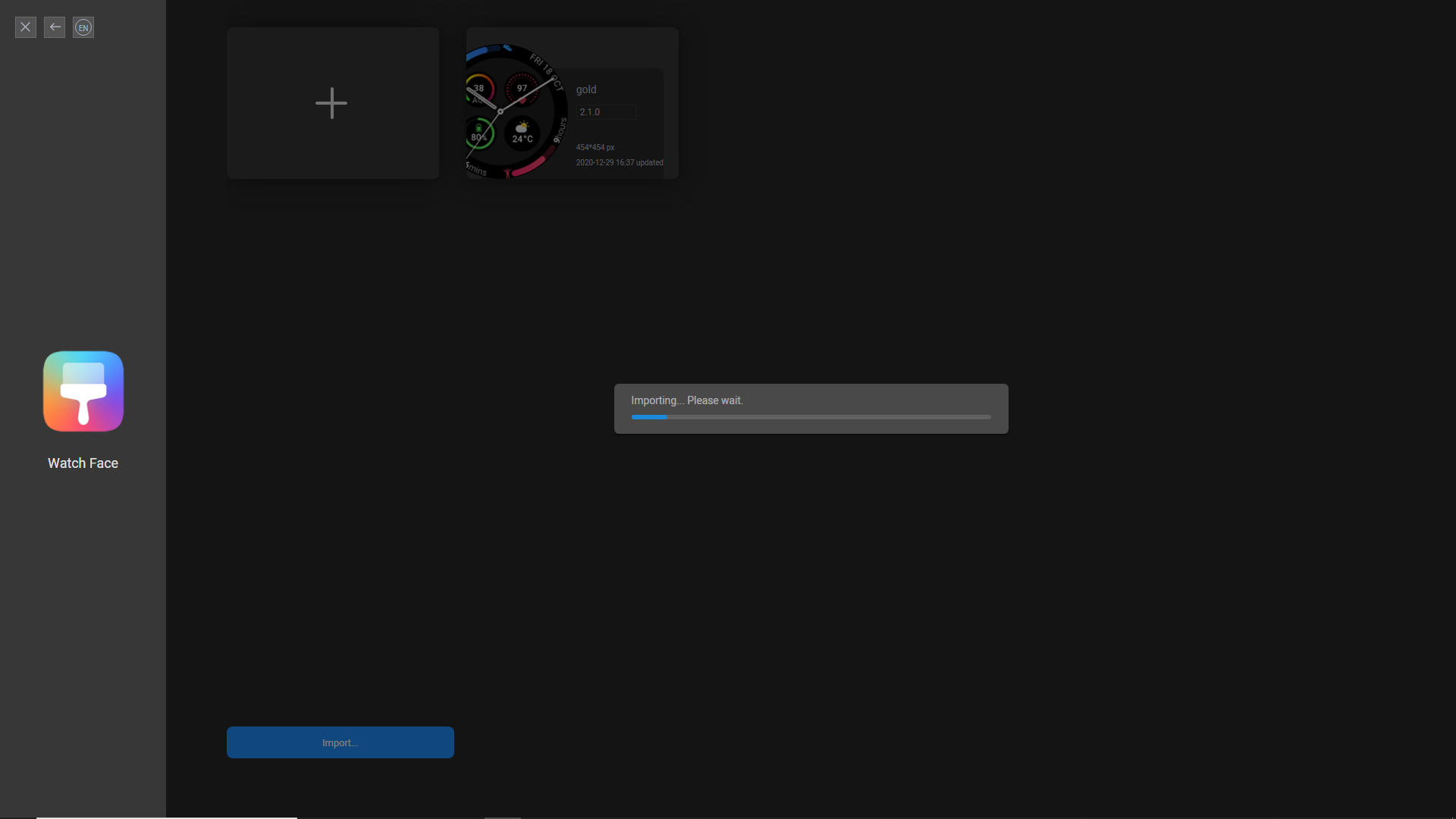